In today’s digital world, password security is more important than ever. Weak or easily guessable passwords are a common cause of data breaches, hacked accounts, and unauthorized access. Fortunately, generating a secure, random password doesn’t have to be complicated—especially if you know a bit of Python!
In this post, we’ll be creating a short Python script that generates a random password using upper and lowercase letters, digits, and symbols. We’ll walk through the code, explain each step, and discuss why it’s important to follow best password practices.
We’ll create a script that:
- Imports the right libraries for randomness and character sets.
- Creates a pool of alphanumeric characters (uppercase and lowercase letters plus digits).
- Adds a randomly selected symbol to increase complexity.
- Ensures your new password is unique (no duplicates).
By the end, you’ll have a clear understanding of how each piece of this script works and how you can adapt it for your own needs.
Go ahead and open VS Code, and create a new Python file. Now, our first 2 lines of code are going to be importing libraries.
Step 1: Importing the Necessary Libraries
import random
import string
What’s happening here?
random
: This Python module lets us generate random numbers, shuffle sequences, and pick random items from lists or strings.string
: This module provides convenient string constants, such asstring.ascii_letters
,string.digits
, andstring.punctuation
.
Why are these imports required?
- We need a randomness source to ensure our passwords can’t be predicted.
- We want easy access to letters, digits, and symbols without manually typing them out.
Step 2: Maintaining a Global Set of Generated Passwords
generated_passwords = set()
What’s happening here?
- We create a set called generated_passwords. In Python, sets are collections of unique items ensuring no duplicates are allowed.
- Every time we generate a password, the script will add it to this set. If we ever generate the same password twice, the script will know and pick a new one instead.
Why is this important?
- Maintaining a set ensures password uniqueness. If you’re generating many passwords in one session, you don’t want to accidentally reuse the same one.
Step 3: Defining the generate_password()
Function
def generate_password():
What’s happening here?
- We declare a function named
generate_passwords()
that encapsulates all the logic for creating a secure password. - It’s helpful to keep our password generation code self-contained, so it’s easy to call wherever needed.
Why is this important?
- Functions make code modular and maintainable. If you want to tweak your password rules later (e.g., change the length or the character sets), you only have to update this one function.
Step 4: Creating Character Pools
Inside the generate_password()
function, the first lines look like this:
alphanumerical_characters = string.ascii_letters + string.digits
symbols = string.punctuation
What’s happening here?
string.ascii_letters
gives us all lowercase (a-z) and uppercase (A-Z) letters.strings.digits
gives us the digits 0-9.- By concatenating them (+), we form a single string that contains both letters and digits (e.g.,
"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
). - string.punctuation gives us symbols (e.g.,
!@#$%^&*()_+-=[]{}|;':",.<>/?
).
Why is this important?
- We’re ensuring we have a robust set of characters to create complex passwords that are harder to guess.
- Separating alphanumerical characters from symbols makes it easy to control how many of each type we add.
Step 5: Generating 8 Random Alphanumeric Characters
password = ''.join(random.choices(alphanumerical_characters, k=8))
What’s happening here?
random.choices(alphanumerical_characters, k=8)
picks 8 random characters (each one could be a letter or digit).''.join(...)
combines those 8 characters into a single string.
Why is this important?
- Having 8 random alphanumeric characters helps ensure the password is long enough to resist common brute-force attacks.
- You can easily adjust the
k=8
parameter to make your passwords longer if you want extra security.
Step 6: Adding a Random Symbol
password += random.choice(symbols)
What’s happening here?
- We append a single random symbol from
string.punctuation
to the end of thepassword
string.
Why is this important?
- Adding at least one symbol makes the password more complex. Many systems require special characters to enhance security.
- It ensures the final password always contains something beyond just letters and digits.
Step 7: Shuffling the Characters
password = ''.join(random.sample(password, len(password)))
What’s happening here?
random.sample(password, len(password))
takes all the characters inpassword
and returns them in a random order.- By joining them back together, we get a completely shuffled password.
Why is this important?
- If we just tacked the symbol onto the end, attackers could guess that the last character might be a symbol.
- Shuffling ensures the symbol could be anywhere in the password, making it less predictable.
Step 8: Ensuring Uniqueness
if password in generated_passwords:
return generate_password()
else:
generated_passwords.add(password)
return password
What’s happening here?
- We check if the newly created password is already in our generated_passwords set.
- If yes, we recursively call
generate_password()
again to generate a new one. - If no, we add the password to generated_passwords and return it.
Why is this important?
- It guarantees that no two generated passwords will be exactly the same during the runtime of the program.
- While collisions are statistically unlikely (especially with strong randomness), this logic adds an extra layer of assurance for uniqueness.
Step 9: Putting It All Together and Printing the Password
print(generated_password())
What’s happening here?
- We call generate_password() to create a new, random password based on our rules.
- We then output (print) the password to the console.
Finally, we have written our Python Script! Name your file but for simplicity sake, I have chosen “password_gen.py”, now choose to save somewhere, I chose the desktop. Now navigate to your Command prompt/Terminal and type the following command.
For myself, I have chose to save the .py file to my Desktop, so we need to navigate to that. Type in cd Desktop.
Once in the Desktop’s Directory, type in the following command: python password_gen.py
Note: Since I am currently utilizing MacOS, it will look slightly different. The command would be python3 instead of just python.
If successful, you should see a 8 alphanumerical character password!
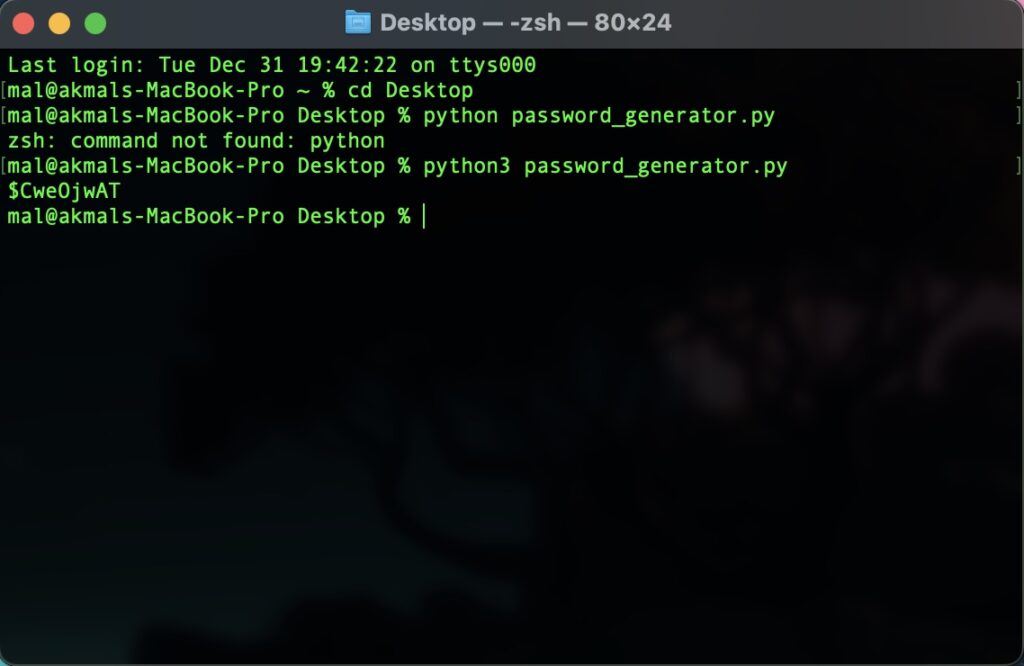